728x90
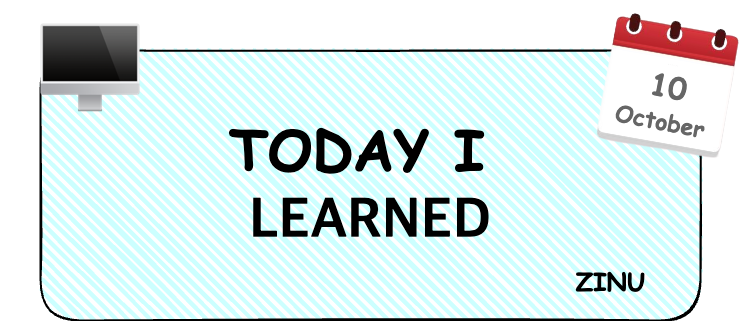
개요
프로젝트에서 결제 시스템을 토스 페이먼츠를 사용하고있습니다.
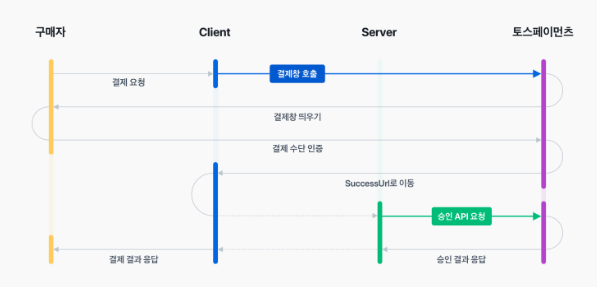
토스 페이먼츠에서는 위와 같이 결제 요청 ➡️ 결제창 호출 ➡️ 승인 API 요청 흐름으로 진행됩니다. 따라서 최종 결제 승인 요청을 할 때에는 토스 페이먼츠에게 API를 요청해야 합니다.
PaymentSuccessDto result = null;
// 최종 결제 승인 요청 URL : config에서 작성한 https://api.tosspayments.com/v1/payments/ + paymentKey
result = restTemplate.postForObject(TossPaymentConfig.URL + paymentKey,
new HttpEntity<>(params,headers),
PaymentSuccessDto.class);
따라서 위와 같이 RestTemplate의 postForObject 메서드를 사용하여 API요청을 했습니다. 본 글에서는 postForObject를 포함해서 RESTful 웹 서비스와의 통신을 간편하게 처리할 수 있도록 다양한 메서드를 알아보겠습니다.
RestTemplate restTemplate = new RestTemplate();
String url = "https://example.com/api/resource";
GET
getForObject(url, responseType)
// GET 요청
MyResponseDto getResponse = restTemplate.getForObject(url, MyResponseDto.class);
- 지정된 URL에서 HTTP GET 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다.
getForEntity(url, responseType)
// GET 요청
ResponseEntity<MyResponseDto> getResponseEntity = restTemplate.getForEntity(url, MyResponseDto.class);
- 지정된 URL에서 HTTP GET 요청을 보내고, 응답을 ResponseEntity로 받아 본문, 상태 코드, 헤더를 확인할 수 있습니다.
POST
postForObject(url, responseType)
// POST 요청
MyRequestDto postRequest = new MyRequestDto("example", 123);
MyResponseDto postResponse = restTemplate.postForObject(url, postRequest, MyResponseDto.class);
- 지정된 URL에 HTTP POST 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다.
postForEntity(url, responseType)
// POST 요청
ResponseEntity<MyResponseDto> postResponseEntity = restTemplate.postForEntity(url, postRequest, MyResponseDto.class);
- URL에 POST 요청을 보내고, 응답을 ResponseEntity로 반환합니다.
PUT
// PUT 요청
MyRequestDto putRequest = new MyRequestDto("updatedExample", 456);
restTemplate.put(url, putRequest);
- 지정된 URL에 HTTP PUT 요청을 보내어 자원을 업데이트합니다.
DELETE
// DELETE 요청
restTemplate.delete(url);
- 지정된 URL에 HTTP DELETE 요청을 보내어 자원을 삭제합니다. 응답 본문은 없고, 상태 코드만 반환됩니다.
HEAD 요청 (헤더만 받기)
// HEAD 요청
HttpHeaders headers = restTemplate.headForHeaders(url);
- 지정된 URL에 HTTP HEAD 요청을 보내고, 응답의 헤더만 반환합니다.
본문은 포함되지 않습니다.
정리
GET | getForObject | URL에서 GET 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다. |
getForEntity | URL에서 GET 요청을 보내고, 응답을 ResponseEntity로 반환합니다. | |
headForHeaders | URL에 HEAD 요청을 보내고, 응답 헤더만 반환합니다. | |
optionsForAllow | URL에 OPTIONS 요청을 보내고, 서버가 지원하는 메서드를 반환합니다. | |
POST | postForObject | URL에 POST 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다. |
postForEntity | URL에 POST 요청을 보내고, 응답을 ResponseEntity로 반환합니다. | |
exchange | 동적으로 요청 메서드(POST 포함)를 설정하고 요청할 수 있습니다. | |
PUT | put | URL에 PUT 요청을 보내어 자원을 업데이트합니다. |
exchange | 동적으로 요청 메서드(PUT 포함)를 설정하고 요청할 수 있습니다. | |
DELETE | delete | URL에 DELETE 요청을 보내어 자원을 삭제합니다. |
exchange | 동적으로 요청 메서드(DELETE 포함)를 설정하고 요청할 수 있습니다. | |
PATCH | exchange | PATCH 요청을 보낼 때 exchange 메서드를 사용하여 자원을 부분적으로 업데이트할 수 있습니다. |
'TIL,일일 회고' 카테고리의 다른 글
[TIL, 일일 회고] 2024.10.12 - @SneakyThrows란❓ (0) | 2024.10.12 |
---|---|
[TIL, 일일 회고] 2024.10.11 - SDK란 무엇일까❓ (토스페이먼츠 SDK) (2) | 2024.10.11 |
[TIL, 일일 회고] 2024.10.09 - @Positive, @PositiveOrZero (0) | 2024.10.09 |
[TIL, 일일 회고] 2024.10.08 - @ComponentScan (0) | 2024.10.08 |
[TIL, 일일 회고] 2024.10.07 - @ModelAttribute가 자동 변환 할 수 없는 타입 (0) | 2024.10.07 |
728x90
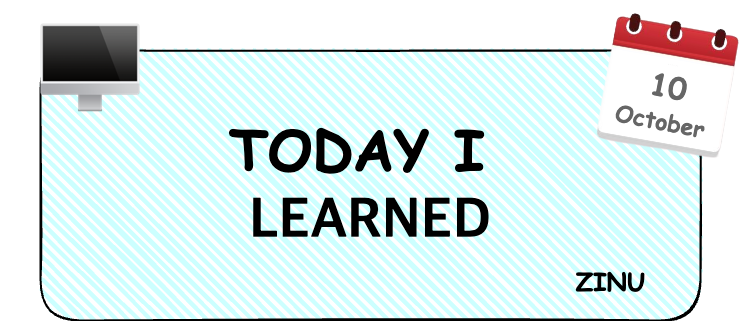
개요
프로젝트에서 결제 시스템을 토스 페이먼츠를 사용하고있습니다.
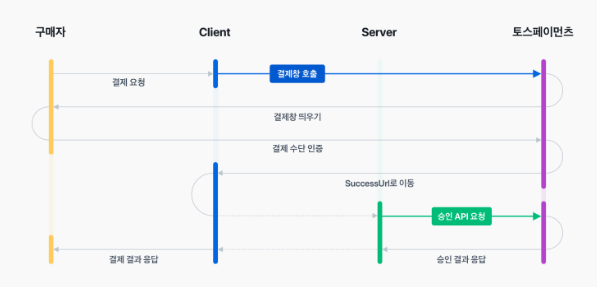
토스 페이먼츠에서는 위와 같이 결제 요청 ➡️ 결제창 호출 ➡️ 승인 API 요청 흐름으로 진행됩니다. 따라서 최종 결제 승인 요청을 할 때에는 토스 페이먼츠에게 API를 요청해야 합니다.
PaymentSuccessDto result = null;
// 최종 결제 승인 요청 URL : config에서 작성한 https://api.tosspayments.com/v1/payments/ + paymentKey
result = restTemplate.postForObject(TossPaymentConfig.URL + paymentKey,
new HttpEntity<>(params,headers),
PaymentSuccessDto.class);
따라서 위와 같이 RestTemplate의 postForObject 메서드를 사용하여 API요청을 했습니다. 본 글에서는 postForObject를 포함해서 RESTful 웹 서비스와의 통신을 간편하게 처리할 수 있도록 다양한 메서드를 알아보겠습니다.
RestTemplate restTemplate = new RestTemplate();
String url = "https://example.com/api/resource";
GET
getForObject(url, responseType)
// GET 요청
MyResponseDto getResponse = restTemplate.getForObject(url, MyResponseDto.class);
- 지정된 URL에서 HTTP GET 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다.
getForEntity(url, responseType)
// GET 요청
ResponseEntity<MyResponseDto> getResponseEntity = restTemplate.getForEntity(url, MyResponseDto.class);
- 지정된 URL에서 HTTP GET 요청을 보내고, 응답을 ResponseEntity로 받아 본문, 상태 코드, 헤더를 확인할 수 있습니다.
POST
postForObject(url, responseType)
// POST 요청
MyRequestDto postRequest = new MyRequestDto("example", 123);
MyResponseDto postResponse = restTemplate.postForObject(url, postRequest, MyResponseDto.class);
- 지정된 URL에 HTTP POST 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다.
postForEntity(url, responseType)
// POST 요청
ResponseEntity<MyResponseDto> postResponseEntity = restTemplate.postForEntity(url, postRequest, MyResponseDto.class);
- URL에 POST 요청을 보내고, 응답을 ResponseEntity로 반환합니다.
PUT
// PUT 요청
MyRequestDto putRequest = new MyRequestDto("updatedExample", 456);
restTemplate.put(url, putRequest);
- 지정된 URL에 HTTP PUT 요청을 보내어 자원을 업데이트합니다.
DELETE
// DELETE 요청
restTemplate.delete(url);
- 지정된 URL에 HTTP DELETE 요청을 보내어 자원을 삭제합니다. 응답 본문은 없고, 상태 코드만 반환됩니다.
HEAD 요청 (헤더만 받기)
// HEAD 요청
HttpHeaders headers = restTemplate.headForHeaders(url);
- 지정된 URL에 HTTP HEAD 요청을 보내고, 응답의 헤더만 반환합니다.
본문은 포함되지 않습니다.
정리
GET | getForObject | URL에서 GET 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다. |
getForEntity | URL에서 GET 요청을 보내고, 응답을 ResponseEntity로 반환합니다. | |
headForHeaders | URL에 HEAD 요청을 보내고, 응답 헤더만 반환합니다. | |
optionsForAllow | URL에 OPTIONS 요청을 보내고, 서버가 지원하는 메서드를 반환합니다. | |
POST | postForObject | URL에 POST 요청을 보내고, 응답 본문을 객체로 변환하여 반환합니다. |
postForEntity | URL에 POST 요청을 보내고, 응답을 ResponseEntity로 반환합니다. | |
exchange | 동적으로 요청 메서드(POST 포함)를 설정하고 요청할 수 있습니다. | |
PUT | put | URL에 PUT 요청을 보내어 자원을 업데이트합니다. |
exchange | 동적으로 요청 메서드(PUT 포함)를 설정하고 요청할 수 있습니다. | |
DELETE | delete | URL에 DELETE 요청을 보내어 자원을 삭제합니다. |
exchange | 동적으로 요청 메서드(DELETE 포함)를 설정하고 요청할 수 있습니다. | |
PATCH | exchange | PATCH 요청을 보낼 때 exchange 메서드를 사용하여 자원을 부분적으로 업데이트할 수 있습니다. |
'TIL,일일 회고' 카테고리의 다른 글
[TIL, 일일 회고] 2024.10.12 - @SneakyThrows란❓ (0) | 2024.10.12 |
---|---|
[TIL, 일일 회고] 2024.10.11 - SDK란 무엇일까❓ (토스페이먼츠 SDK) (2) | 2024.10.11 |
[TIL, 일일 회고] 2024.10.09 - @Positive, @PositiveOrZero (0) | 2024.10.09 |
[TIL, 일일 회고] 2024.10.08 - @ComponentScan (0) | 2024.10.08 |
[TIL, 일일 회고] 2024.10.07 - @ModelAttribute가 자동 변환 할 수 없는 타입 (0) | 2024.10.07 |