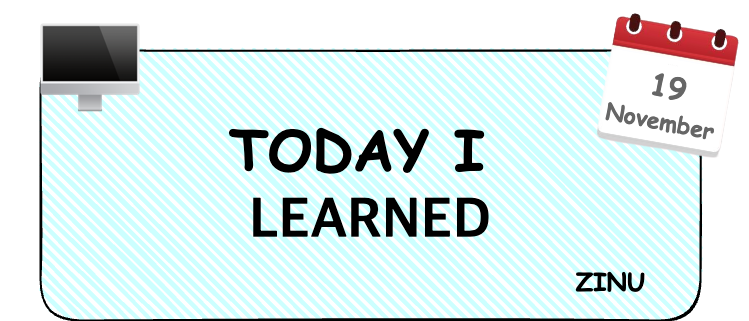
개요
"백준 - 팩토리얼 0의 개수" 문제를 풀다가 BigInteger의 길이를 계산해야 했습니다. 이 문제에서는 매우 큰 수의 팩토리얼을 계산한 후, 그 수에서 뒤에 붙은 0의 개수를 구하는 문제가 주어집니다.
이때, 팩토리얼의 값은 매우 커질 수 있으므로 int나 long으로는 처리가 불가능하고, BigInteger 클래스를 사용해야 합니다.
BigInteger는 매우 큰 숫자를 다룰 수 있는 클래스이지만, 숫자의 길이를 구하는 메서드는 기본적으로 제공되지 않습니다.
따라서 BigInteger의 길이를 계산하려면 몇 가지 방법을 사용해야 합니다. 이번 포스팅에서는 BigInteger 객체의 길이를 구하는 방법을 정리하고자 합니다.
문자열로 변환 후 길이 구하기
BigInteger의 길이를 계산하는 가장 간단한 방법은 BigInteger 객체를 문자열로 변환한 후, 그 문자열의 길이를 계산하는 것입니다. 숫자를 문자열로 바꾸면, 숫자의 자릿수를 쉽게 확인할 수 있기 때문입니다.
예시 코드
import java.math.BigInteger;
public class BigIntegerLength {
public static void main(String[] args) {
// BigInteger 객체 생성
BigInteger bigInt = new BigInteger("1234567890123456789012345678901234567890");
// BigInteger를 문자열로 변환하여 길이 구하기
String str = bigInt.toString();
int length = str.length();
// 길이 출력
System.out.println("BigInteger의 길이: " + length);
}
}
BigInteger의 길이: 40
- bigInt는 1234567890123456789012345678901234567890라는 큰 수를 담고 있습니다.
- toString() 메서드를 통해 BigInteger를 문자열로 변환한 뒤, length() 메서드를 사용하여 문자열의 길이를 구합니다.
- 이 경우, 40자리 숫자이므로 출력값은 40입니다.
백준 - 팩토리얼 0의 개수
import java.math.BigInteger;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int N = sc.nextInt();
BigInteger factorialNumber = factorial(N); // BigInteger로 팩토리얼 계산
int cnt = 0;
// 팩토리얼을 문자열로 바꾸고 끝에서부터 '0'을 세기
String factorialString = factorialNumber.toString();
for (int i = factorialString.length() - 1; i >= 0; i--) {
if(factorialString.charAt(i) == '0'){ // '0' 문자와 비교
cnt++;
} else {
break; // '0'이 아닌 숫자가 나오면 종료
}
}
System.out.println(cnt);
}
// BigInteger로 팩토리얼 계산
private static BigInteger factorial(int n){
BigInteger result = BigInteger.ONE;
for (int i = 2; i <= n; i++) {
result = result.multiply(BigInteger.valueOf(i)); // BigInteger로 곱셈
}
return result;
}
}
'Coding Test > 백준' 카테고리의 다른 글
[백준, 1789번] 수들의 합 (구현, 그리디 알고리즘, Java) (0) | 2024.11.22 |
---|---|
[백준, 1475번] 방 번호 (구현, Java) (0) | 2024.11.21 |
[백준, 10815번] 숫자 카드 (정렬, 이진 탐색, Java) (0) | 2024.11.19 |
[백준, 11050번] 이항 계수 1 (수학, 구현, 조합론, Java) (1) | 2024.11.18 |
[백준, 1296번] 팀 이름 정하기 (구현, 문자열, 정렬, HashMap, Java) (0) | 2024.11.16 |
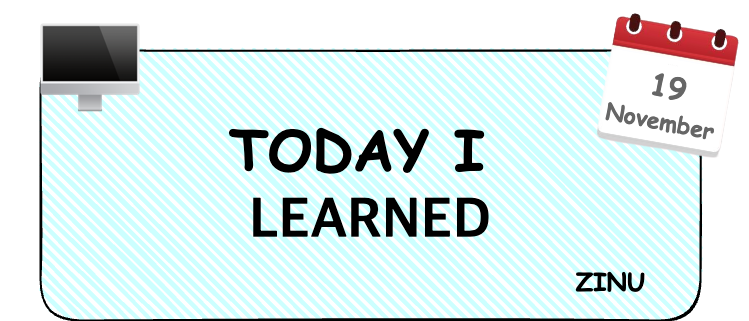
개요
"백준 - 팩토리얼 0의 개수" 문제를 풀다가 BigInteger의 길이를 계산해야 했습니다. 이 문제에서는 매우 큰 수의 팩토리얼을 계산한 후, 그 수에서 뒤에 붙은 0의 개수를 구하는 문제가 주어집니다.
이때, 팩토리얼의 값은 매우 커질 수 있으므로 int나 long으로는 처리가 불가능하고, BigInteger 클래스를 사용해야 합니다.
BigInteger는 매우 큰 숫자를 다룰 수 있는 클래스이지만, 숫자의 길이를 구하는 메서드는 기본적으로 제공되지 않습니다.
따라서 BigInteger의 길이를 계산하려면 몇 가지 방법을 사용해야 합니다. 이번 포스팅에서는 BigInteger 객체의 길이를 구하는 방법을 정리하고자 합니다.
문자열로 변환 후 길이 구하기
BigInteger의 길이를 계산하는 가장 간단한 방법은 BigInteger 객체를 문자열로 변환한 후, 그 문자열의 길이를 계산하는 것입니다. 숫자를 문자열로 바꾸면, 숫자의 자릿수를 쉽게 확인할 수 있기 때문입니다.
예시 코드
import java.math.BigInteger;
public class BigIntegerLength {
public static void main(String[] args) {
// BigInteger 객체 생성
BigInteger bigInt = new BigInteger("1234567890123456789012345678901234567890");
// BigInteger를 문자열로 변환하여 길이 구하기
String str = bigInt.toString();
int length = str.length();
// 길이 출력
System.out.println("BigInteger의 길이: " + length);
}
}
BigInteger의 길이: 40
- bigInt는 1234567890123456789012345678901234567890라는 큰 수를 담고 있습니다.
- toString() 메서드를 통해 BigInteger를 문자열로 변환한 뒤, length() 메서드를 사용하여 문자열의 길이를 구합니다.
- 이 경우, 40자리 숫자이므로 출력값은 40입니다.
백준 - 팩토리얼 0의 개수
import java.math.BigInteger;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int N = sc.nextInt();
BigInteger factorialNumber = factorial(N); // BigInteger로 팩토리얼 계산
int cnt = 0;
// 팩토리얼을 문자열로 바꾸고 끝에서부터 '0'을 세기
String factorialString = factorialNumber.toString();
for (int i = factorialString.length() - 1; i >= 0; i--) {
if(factorialString.charAt(i) == '0'){ // '0' 문자와 비교
cnt++;
} else {
break; // '0'이 아닌 숫자가 나오면 종료
}
}
System.out.println(cnt);
}
// BigInteger로 팩토리얼 계산
private static BigInteger factorial(int n){
BigInteger result = BigInteger.ONE;
for (int i = 2; i <= n; i++) {
result = result.multiply(BigInteger.valueOf(i)); // BigInteger로 곱셈
}
return result;
}
}
'Coding Test > 백준' 카테고리의 다른 글
[백준, 1789번] 수들의 합 (구현, 그리디 알고리즘, Java) (0) | 2024.11.22 |
---|---|
[백준, 1475번] 방 번호 (구현, Java) (0) | 2024.11.21 |
[백준, 10815번] 숫자 카드 (정렬, 이진 탐색, Java) (0) | 2024.11.19 |
[백준, 11050번] 이항 계수 1 (수학, 구현, 조합론, Java) (1) | 2024.11.18 |
[백준, 1296번] 팀 이름 정하기 (구현, 문자열, 정렬, HashMap, Java) (0) | 2024.11.16 |